Java File I/O (NIO.2) is part of the Oracle Certified Professional (OCP) Java 7 Certification exam.
The basic classes to focus in java.nio.file are:
- Path and Paths
- FileSystem and FileSystems
- Files and FileStore
The 1Z0-805 upgrade exam (Beta exam was 1Z1-805) objectives for NIO.2 are:
1. Use the Path class to operate on file and directory paths
Path object can be created using Paths.get(...) or FileSystem.getPath(...). FileSystem object can be created from FileSystems.
Path has methods to operate on the path: getFileName(), getName(idx), getNameCount(), getParent(), getRoot(), hashCode(), isAbsolute(), iterator(), normalize(), subpath(begin,end), toFile() - returns java.io.File, toString()
Path has methods to operate based on another given Path/String: startsWith, endsWith, relativize, resolve, resolveSibling, comparesTo
Path has 3 ways to convert a path toAbsolutePath(), toUri(), toRealPath(Link Option). toRealPath(...) returns an absolute path after resolving redundancies and if argument is true then resolving symbolic links as well.
register method can be used to register a Path for a WatchService and returns a WatchKey.
Exam Watch:
- Path is system dependent. Path on Windows and Linux with same directory structure will be different.
- Path representing file/dir may not exist
- Path can be operated on to append, extract pieces, duplicate, or compare, but java.nio.file.File has to be used on the Path for actual file operations
2. Use the Files class to check, delete, copy, or move a file or directory
Files class has a set of static methods to work on a file/dir. Methods in Files are symbolic link aware.
Simple example to create file using NIO.2:
Charset charset = Charset.forName("US-ASCII");
String str = "test"; try (BufferedWriter writer = Files.newBufferedWriter(myFile, charset)) { writer.write(str, 0, str.length()); } catch (IOException ioe) { System.err.format("IOException: %s%n", ioe); }
File I/O Methods complexity diagram:
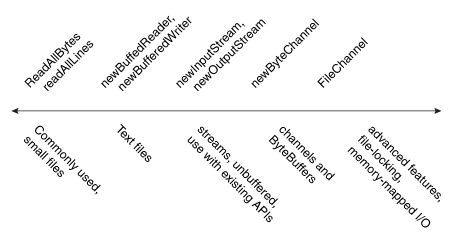
[Ref: Java Tutorials, oracle.com]
Check
Check file: Files.exists(Path, LINK_OPTIONS...), Files.notExists(Path, LINK_OPTIONS...)
If both return false then existence can not be determined - possible when access permission is denied
Check if 2 Paths point to same file: Files.isSameFile(Path, Path)
Delete
Files.delete(Path) may throw NoSuchFileException, DirectoryNotEmptyException, or else for permission problems - IOException.
ExamWatch:
Files.deleteIfExists(Path) will never throw NoSuchFileException
Copy
syntax: Files.copy(Path, Path, COPY_OPTIONS...);example: Files.copy( src, target, REPLACE_EXISTING, NOFOLLOW_LINKS);
COPY_OPTIONS (Requires import static java.nio.file.StandardCopyOption.*;):
- REPLACE_EXISTING - will replace existing file or symbolic link (not target of link) and empty directory. If target is existing non empty directory then FileAlreadyExistsException is thrown.
- COPY_ATTRIBUTES - will copy file attributes including file's last modified time
- NOFOLLOW_LINKS - when specified, the symbolic link will be copied and not the target of the link
Move
syntax: Files.move(Path, Path, MOVE_OPTIONS...);
example: Files.move( src, target, REPLACE_EXISTING, ATOMIC_MOVE);
MOVE_OPTIONS (Requires import static java.nio.file.StandardCopyOption.*;):
- REPLACE_EXISTING
- ATOMIC_MOVE - exception is thrown if filesystem does not support atomic move
Some specific exceptions extend FileSystemException that has useful methods - getFile(), getMessage(), getReason(), getOtherFile() if any other file was involved.
3. Read and change file and directory attributes
Check accessibility with Files: isReadable(Path), isWritable(Path), isExecutable(Path)
Also available in Files:
- size(Path)
- isDirectory(Path, LinkOption), isRegularFile(Path, LinkOption...), isSymbolicLink(Path), isHidden(Path) getLastModifiedTime(Path, LinkOption...), setLastModifiedTime(Path,FileTime)
- getOwner(Path, LinkOption...), setOwner(Path, UserPrincipal)
- getPosixFilePermissions(Path, LinkOption...), setPosixFilePermissions(Path, Set<PosixFilePermission>)
getAttribute(Path, String, LinkOption...)
,setAttribute(Path, String, Object, LinkOption...)
Files.
readAttributes(Path, String, LinkOption...)
or Files.readAttributes(Path, Class<A>, LinkOption...)
PredefinedViews:
BasicFileAttributeView
, DosFileAttributeView
, PosixFileAttributeView, FileOwnerAttributeView, AclFileAttributeView
, UserDefinedFileAttributeView
– Enables support of metadata that is user defined.4. Recursively access a directory tree
Create a
FileVisitor
. Then, either of the following can be used:-
walkFileTree(Path, FileVisitor)
-
walkFileTree(Path, Set<FileVisitOption - FOLLOW_LINK>, int levelsToVisit, FileVisitor)
5. Find a file by using the PathMatcher class
For FileSystem syntax can be "glob" or "regex"
String pattern = ...; PathMatcher matcher = FileSystems.getDefault().getPathMatcher(syntax + ":" + pattern);
glob:
* 0 or more char, but does not cross directory. Will match .<file>
** 0 or more char crossing directories
? single character of name
[] single character or a range. Within [], *?\ will match itself.
{} comma separated subpatterns\ escape for special characters
6. Watch a directory for changes by using WatchService
Steps to create a WatchService:
- Create "watcher", a
WatchService
for the file system.WatchService watcher = FileSystems.getDefault().newWatchService();
- Register each dir to be watched with the
watcher. When registering specify the type of events
for notification. A
WatchKey
instance is received for each registered directory.
Path dir = ...;WatchKey key = dir.register(watcher, ENTRY_DELETE, ENTRY_MODIFY);
- Implement an infinite loop to wait for incoming events. When an event occurs, the key is signaled and placed into the watcher's queue.
- Retrieve the key from the watcher's queue and obtain the file name from the key.
WatchService provides poll(), poll(long, TimeUnit), take() to get a signalled key. - Retrieve each pending event using key.pollEvents() and process using event.kind().
event.context() returns the file name. - Reset the key by calling key.reset(). If true resume waiting for events, else break.
- Close the service: The watch service exits when either the thread exits or when it is closed by calling watcher.close().
[Ref] Java Tutorials on File I/O featuring NIO.2
Java 7 launch video on NIO.2 from Oracle Media Network:
No comments:
Post a Comment